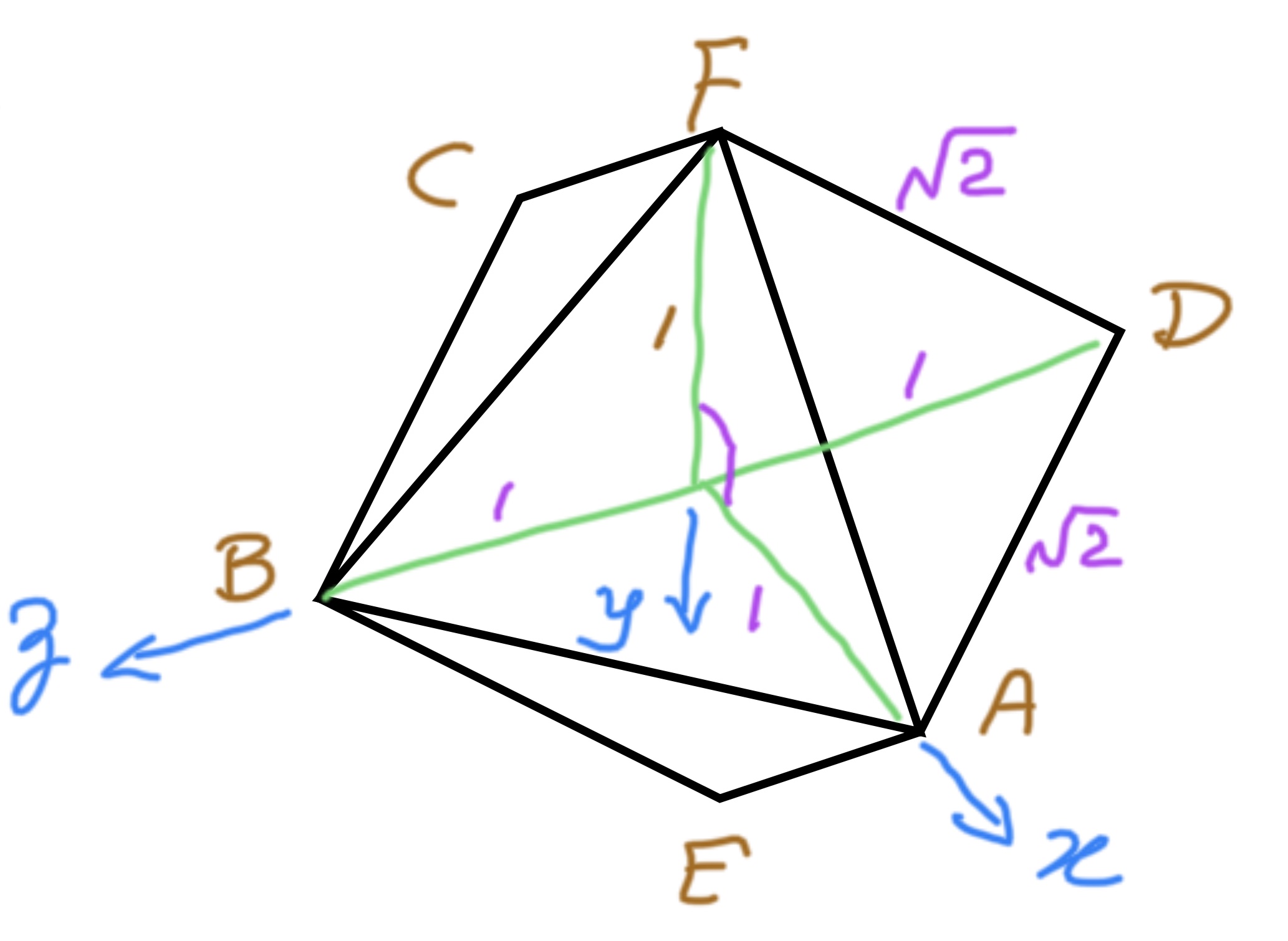
This is what it looks like when finished:
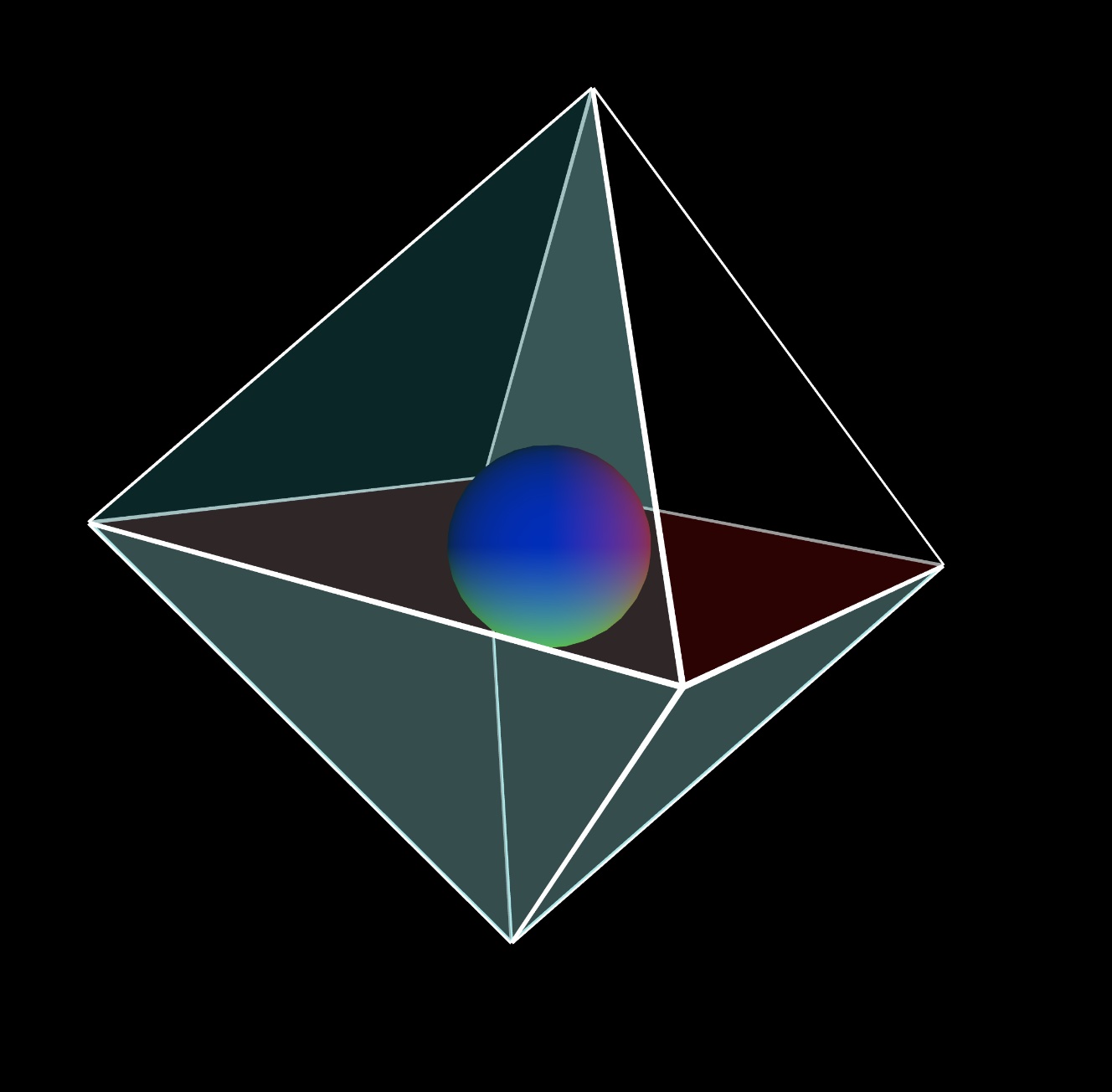
Our goal is to let octahedron ABCDEF spin slowly around its center, which is also the origin of our 3D coordinates space.
draw a sphere centered at origin
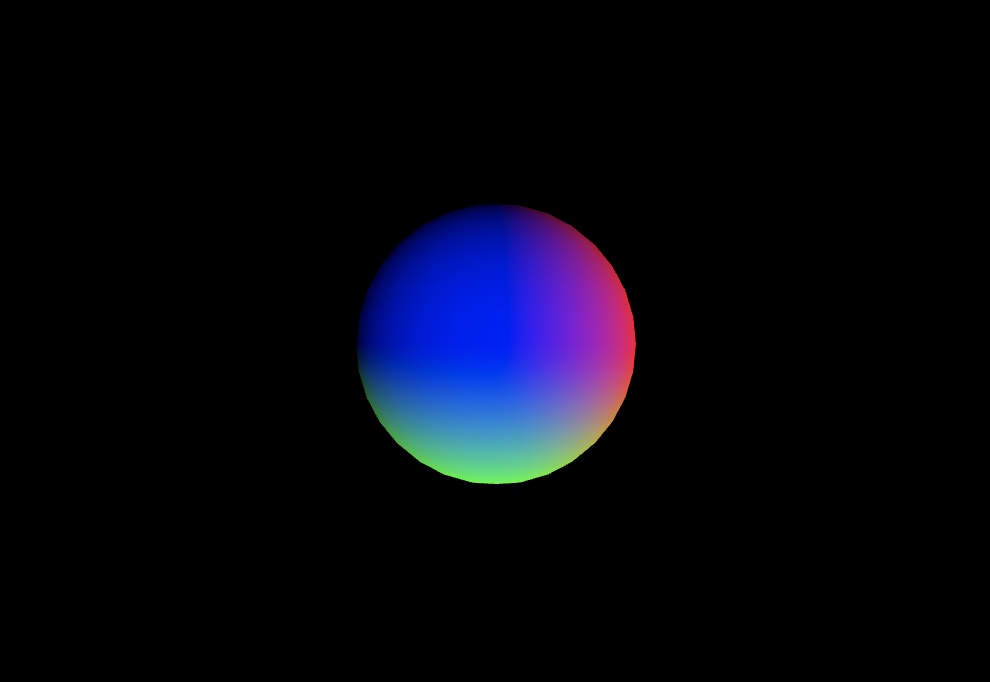
draw the 1st face ABF
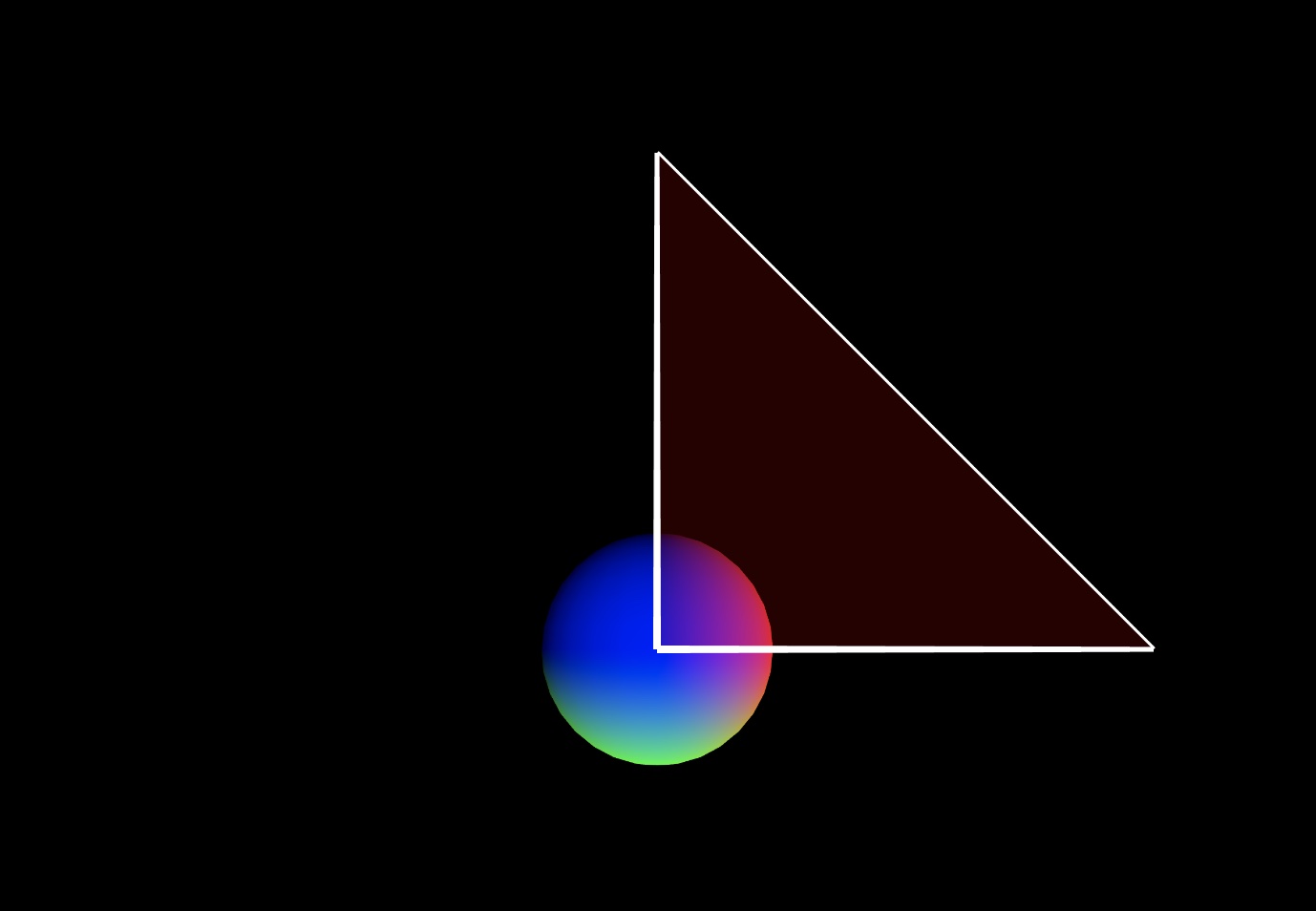
draw the 2nd face BCF
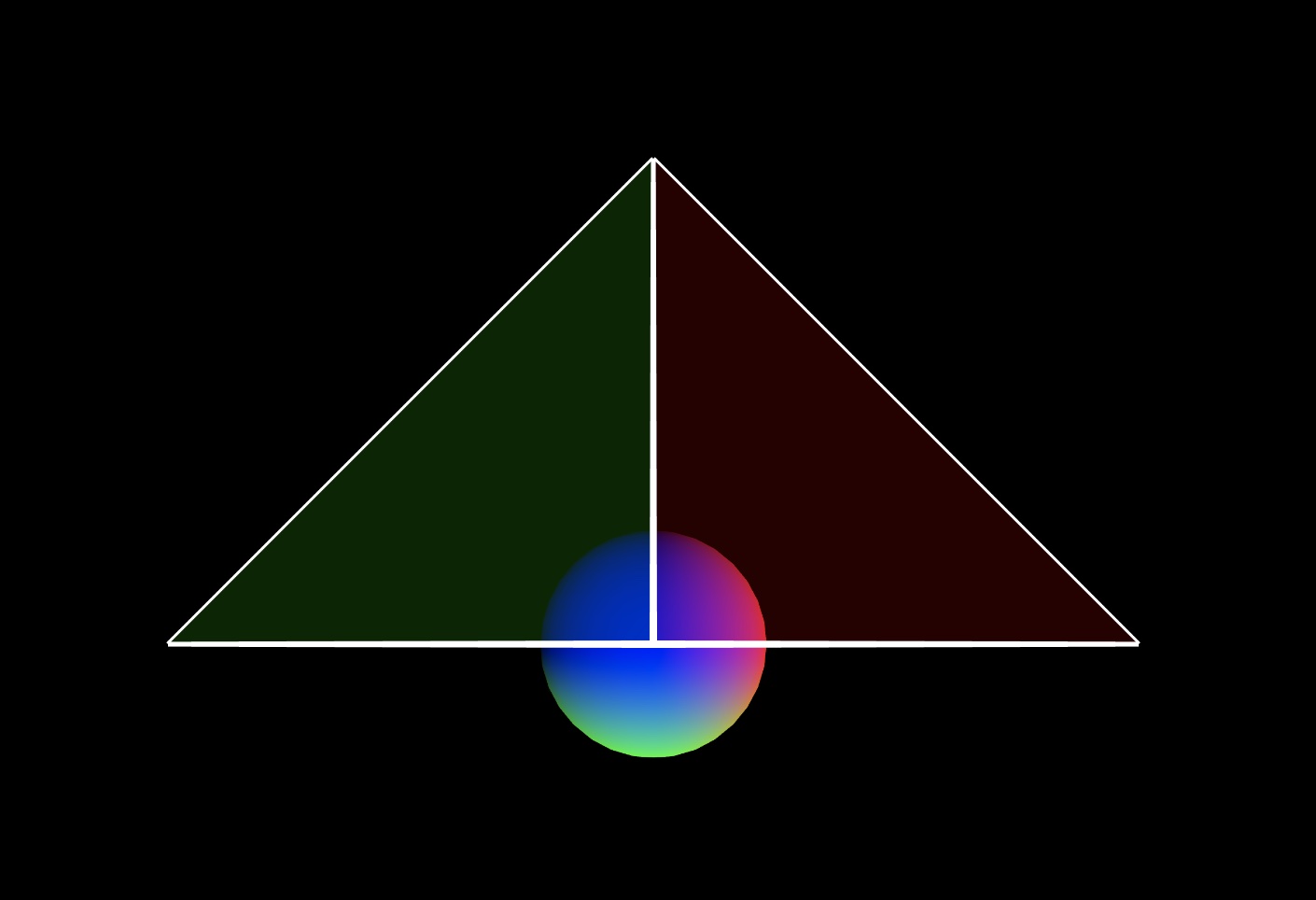
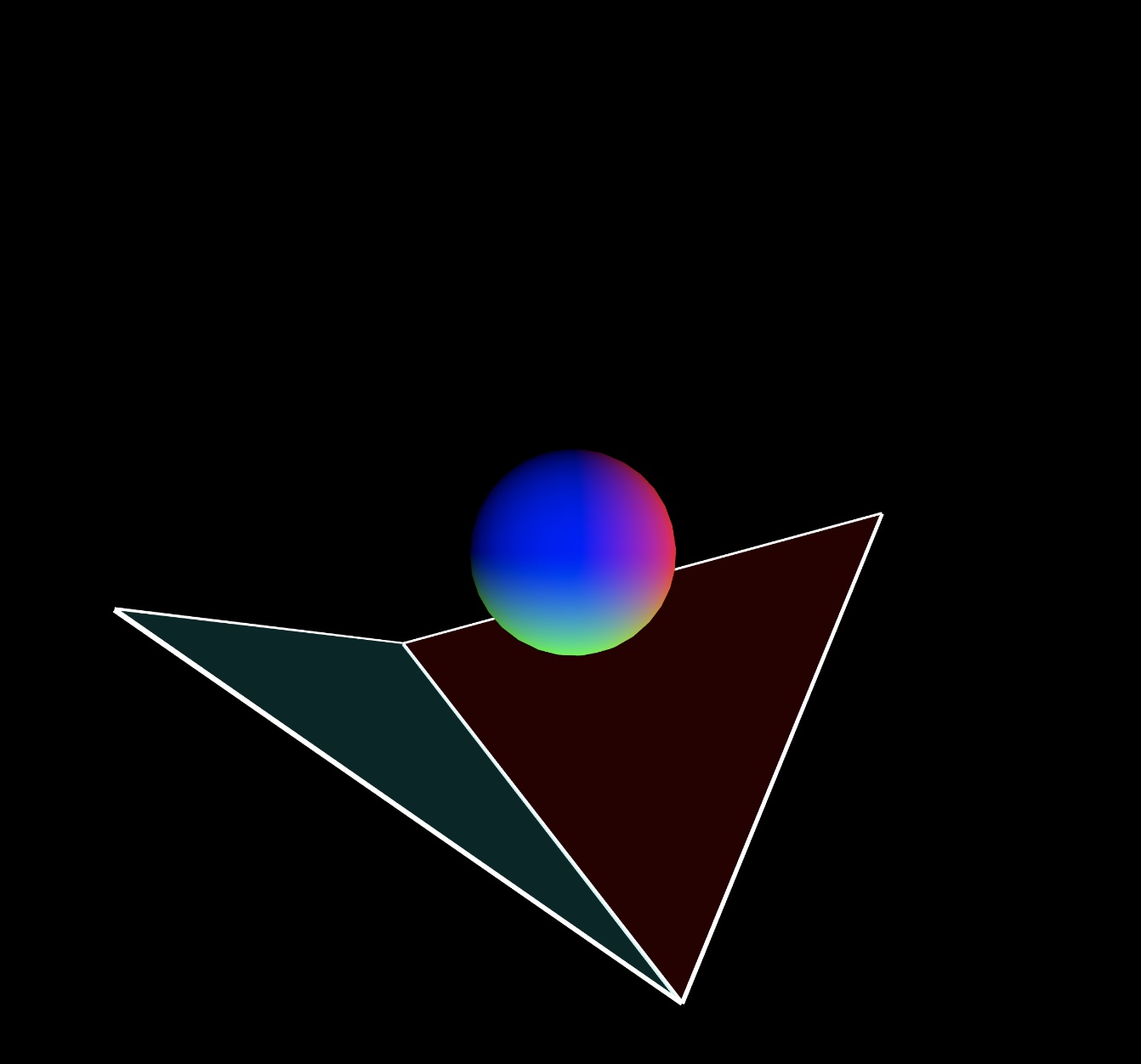
draw the 3rd face CDF
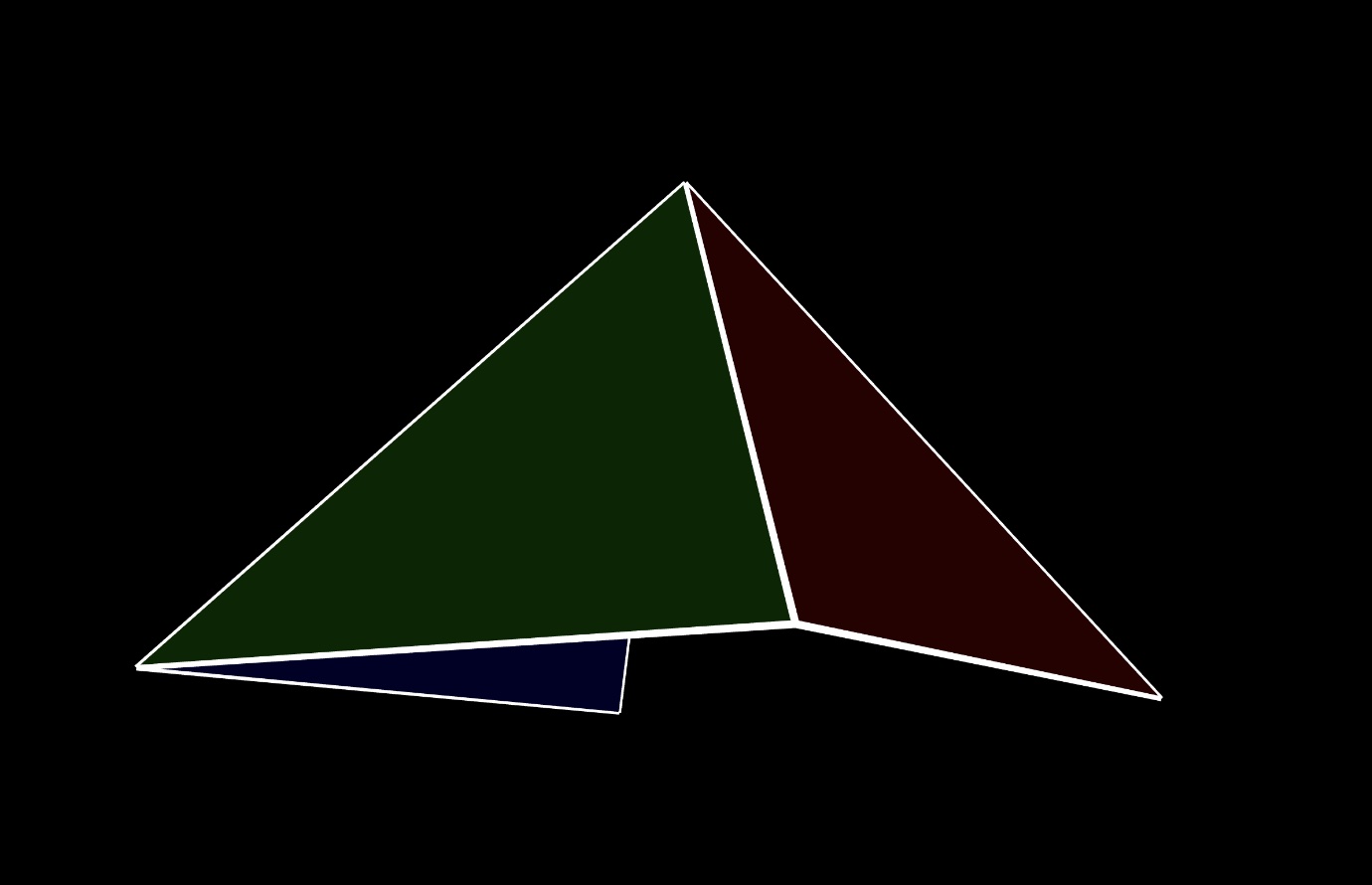
file octahedron.html
<!DOCTYPE html>
<html lang="en">
<head>
<title>Octahedron</title>
<meta name="viewport"
content="width=device-width,
initial-scale=1" />
<script src=
"https://cdnjs.cloudflare.com/
ajax/libs/p5.js/1.5.0/
p5.min.js">
</script>
<style>
canvas {
width: 100% !important;
height: 100% !important;
}
</style>
<meta charset="utf-8" />
</head>
<body>
<p>
Octahedron
</p>
<script src=
"/scripts/octahedron.js">
</script>
</body>
</html>
file octahedron.js
let ang = 0;
let u = 500;
function setup() {
createCanvas(960, 960, WEBGL);
stroke(255);
strokeWeight(3);
}
function draw() {
background(0);
normalMaterial();
rotateY(ang);
rotateX(ang*0.5);
rotateZ(ang*0.2);
strokeWeight(15);
sphere(70);
let a = [ u, 0, 0];
let b = [ 0, 0, u];
let c = [-u, 0, 0];
let d = [ 0, 0, -u];
let e = [ 0, u, 0];
let f = [ 0, -u, 0];
stroke(255);
strokeWeight(3);
triangleShape(a,b,f,200,0,0);
triangleShape(b,c,f,0,200,0);
triangleShape(c,d,f,0,0,200);
triangleShape(d,a,f,200,200,0);
triangleShape(a,b,e,200,0,200);
triangleShape(b,c,e,0,200,200);
triangleShape(c,d,e,0,100,100);
triangleShape(d,a,e,200,200,0);
ang += 0.01;
}
function triangleShape(i,j,k,r,g,b){
fill(r, g, b, 100);
beginShape();
vertex(i[0], i[1], i[2]);
vertex(j[0], j[1], j[2]);
vertex(k[0], k[1], k[2]);
endShape(CLOSE);
}
ch 2.2 The Regular Polyhedra p21 of
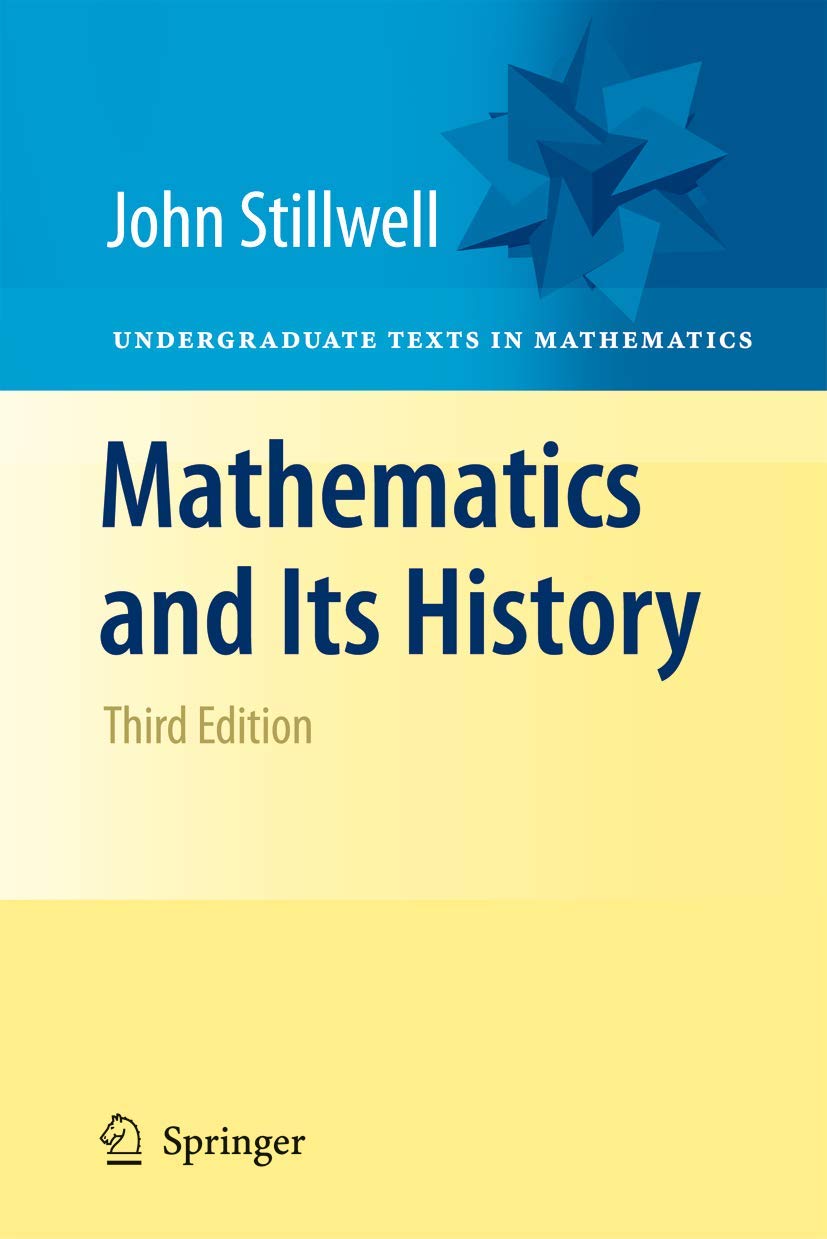