👍 g++ -std=c++11 prt_binary_tree_preorder.cpp
👍 ./a.out
E
D
B
A
*
*
C
*
*
*
H
F
*
G
*
*
*
👍 cat prt_binary_tree.cpp
#include <iostream>
using namespace std;
typedef char Item;
struct node{Item item; node *l, *r;};
typedef node *link;
void printnode(Item x, int h) {
for (int i = 0; i < h; i++)
cout << " ";
cout << x << endl;
}
void show(link t, int h) {
if (t == 0)
{printnode('*', h); return;}
printnode(t->item, h);
show(t->l, h+1);
show(t->r, h+1);
}
int main() {
node a{'A'};
node c{'C'};
node b{'B', &a, &c};
node d{'D', &b};
node g{'G'};
node f{'F', 0, &g};
node h{'H', &f};
node e{'E', &d, &h};
show(&e, 0);
}
5.7 Recursive Binary-Tree Algorithm p251 of
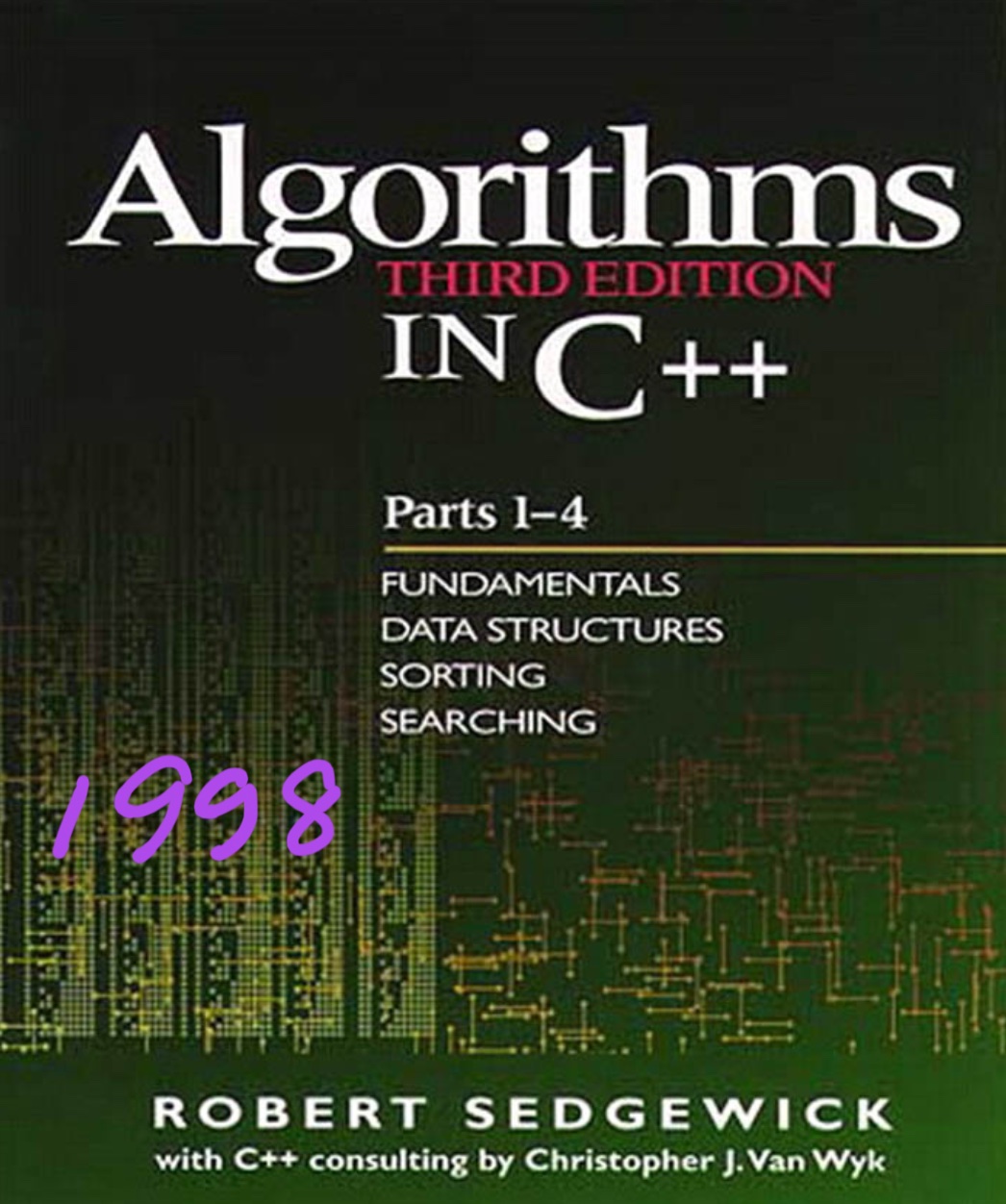