👍 g++ -std=c++11 stl_pq_kids_age.cpp
👍 ./a.out
pqName1:(Ryan,11) (Peter,14) (Felix,12)
pqName2:(Felix,12) (Peter,14) (Ryan,11)
pqAge:(Peter,14) (Felix,12) (Ryan,11)
👍 cat stl_pq_kids_age.cpp
#include <iostream>
#include <vector>
#include <queue>
using namespace std;
struct Kid {
string name;
int age;
bool operator<(const Kid &k) const
{ return name < k.name; }
bool operator==(const Kid &k) const {
return name == k.name &&
age == k.age; }
bool operator>(const Kid &k) const {
return !(*this == k) &&
!(*this < k); }
};
class lesserAge {
public:
bool operator()(const Kid &k1,
const Kid &k2)const
{ return k1.age < k2.age; }
};
ostream& operator<<(ostream &o,
const Kid &k){
o << "(" << k.name << ","
<< k.age << ")";
return o;
}
template<class A, class B, class C>
void prt(string msg,
priority_queue<A,B,C> q) {
cout << msg;
while (!q.empty()) {
cout << q.top() << ' ';
q.pop();
}
cout << endl;
}
int main() {
Kid kids[]{Kid{"Felix", 12},
Kid{"Peter", 14},
Kid{"Ryan", 11}};
priority_queue<Kid> pqName1(kids,
kids+3);
prt("pqName1:", pqName1);
priority_queue<Kid,
vector<Kid>,
greater<Kid>>
pqName2(kids, kids+3);
prt("pqName2:", pqName2);
priority_queue<Kid,
vector<Kid>,
lesserAge>
pqAge(kids, kids+3);
prt("pqAge:", pqAge);
}
ch 4.6 PRIORITY QUEUES IN THE STANDARD TEMPLATE LIBRARY p152 of
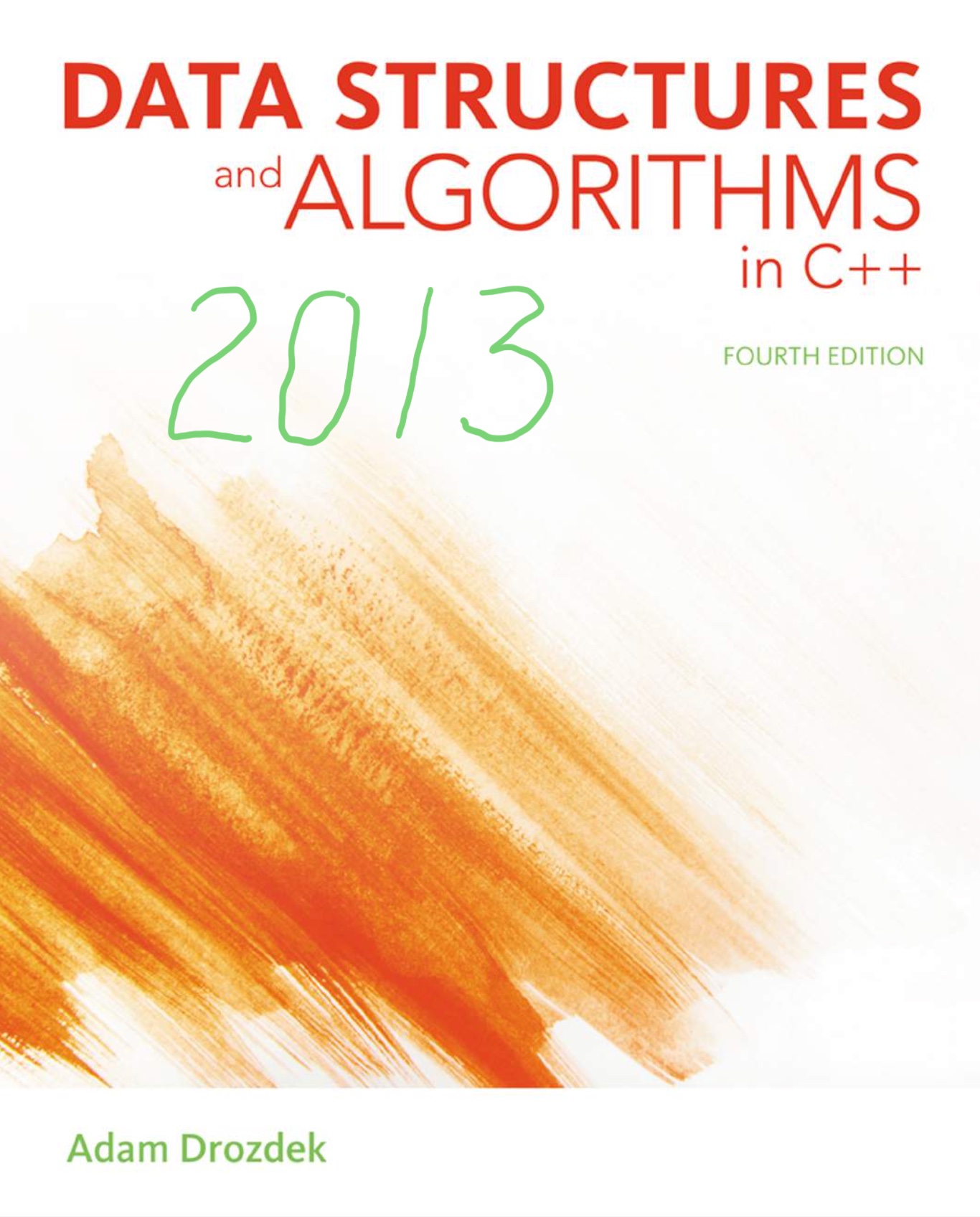